Welcome to an exciting journey into the world of AI agents! In this blog post, we’ll explore how to create a fun and interactive debate simulation between a Data Analyst and a Data Scientist using OpenAI’s GPT models and Streamlit. This application not only showcases the power of AI but also adds a humorous twist to the age-old debate between these two roles.
What You’ll Learn
By the end of this tutorial, you will know how to:
- Set up a multi-agent debate using OpenAI models.
- Add humor and creativity to AI-generated responses.
- Convert text into audio using gTTS and play it back in Streamlit.
- Build a fun, interactive Streamlit app that brings the debate to life with both text and voice.
Setting Up Your Environment
Before diving into the code, ensure you have the required libraries installed. You will need:
- Streamlit – for building the web application.
- OpenAI – to access the GPT models.
- gTTS – for converting text to speech.
- PIL – for image processing.
Make sure to install these libraries using pip:
pip install streamlit openai gtts pillow
Testing Your OpenAI API Key
Before you start coding, it’s important to test if your OpenAI API key works. Create a function to verify the key:
def test_openai_key(api_key):
import openai
openai.api_key = api_key
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content": "Hello!"}]
)
return response.choices[0].message.content
Make sure to replace api_key
with your actual API key.
Understanding AI Agents
In the context of AI and LLMs (Large Language Models), agents refer to distinct roles or personas that simulate different characters during a conversation or task. In our application, we will create two agents:
- Data Analyst – defends their job while throwing playful insults at the Data Scientist.
- Data Scientist – responds to the Data Analyst’s arguments while defending their role.
Creating the Agents
To create our agents, we will define a function that generates responses based on the role assigned to the agent. Here’s how it looks:
def generate_agent_response(role_name, prompt):
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[{"role": "system", "content": f"You are a witty and sarcastic {role_name}. You are here to defend your job while throwing in some insults."},
{"role": "user", "content": prompt}]
)
return response.choices[0].message.content
Building the Debate Logic
Now that we have our agents, we can start building the debate logic. We will run a loop for multiple rounds of debate:
def start_debate():
analyst_prompt = "Explain why being a Data Analyst is the best job compared to a Data Scientist."
data_scientist_prompt = "Explain why being a Data Scientist is the best job compared to a Data Analyst."
for round in range(3):
analyst_response = generate_agent_response("Data Analyst", analyst_prompt)
print(f"Data Analyst: {analyst_response}")
data_scientist_response = generate_agent_response("Data Scientist", data_scientist_prompt)
print(f"Data Scientist: {data_scientist_response}")
analyst_prompt = data_scientist_response
data_scientist_prompt = analyst_response
Building the Streamlit App
Now, let’s integrate everything into a Streamlit app. We will create a simple layout with a button to start the debate:
import streamlit as st
from gtts import gTTS
import os
st.set_page_config(layout="wide")
st.title("Data Analyst vs Data Scientist Debate")
if st.button("Start the Debate"):
start_debate()
Adding Text-to-Speech Functionality
To enhance user experience, we can add a feature to convert the text responses into audio. Here’s how you can implement this:
def text_to_speech(text, filename):
tts = gTTS(text=text, lang='en')
tts.save(filename)
return filename
Then, call this function after generating each response:
audio_file = text_to_speech(analyst_response, "analyst.mp3")
st.audio(audio_file)
Deploying the Streamlit App
Once you have everything working locally, you can deploy your Streamlit app using Streamlit Community Cloud. Follow these steps:
- Create a new GitHub repository and push your code.
- Navigate to Streamlit Community Cloud, click on “New app”.
- Select your repository, branch, and the main file of your app.
- Click “Deploy”.
Conclusion
Congratulations! You’ve successfully built an interactive AI debate simulation using OpenAI’s GPT models and Streamlit. This project demonstrates the capabilities of AI agents in generating dynamic content and how to create engaging applications.
Feel free to experiment with adding more agents or enhancing the debate structure. If you have any questions or suggestions, let me know in the comments below!
Happy coding! 🎈
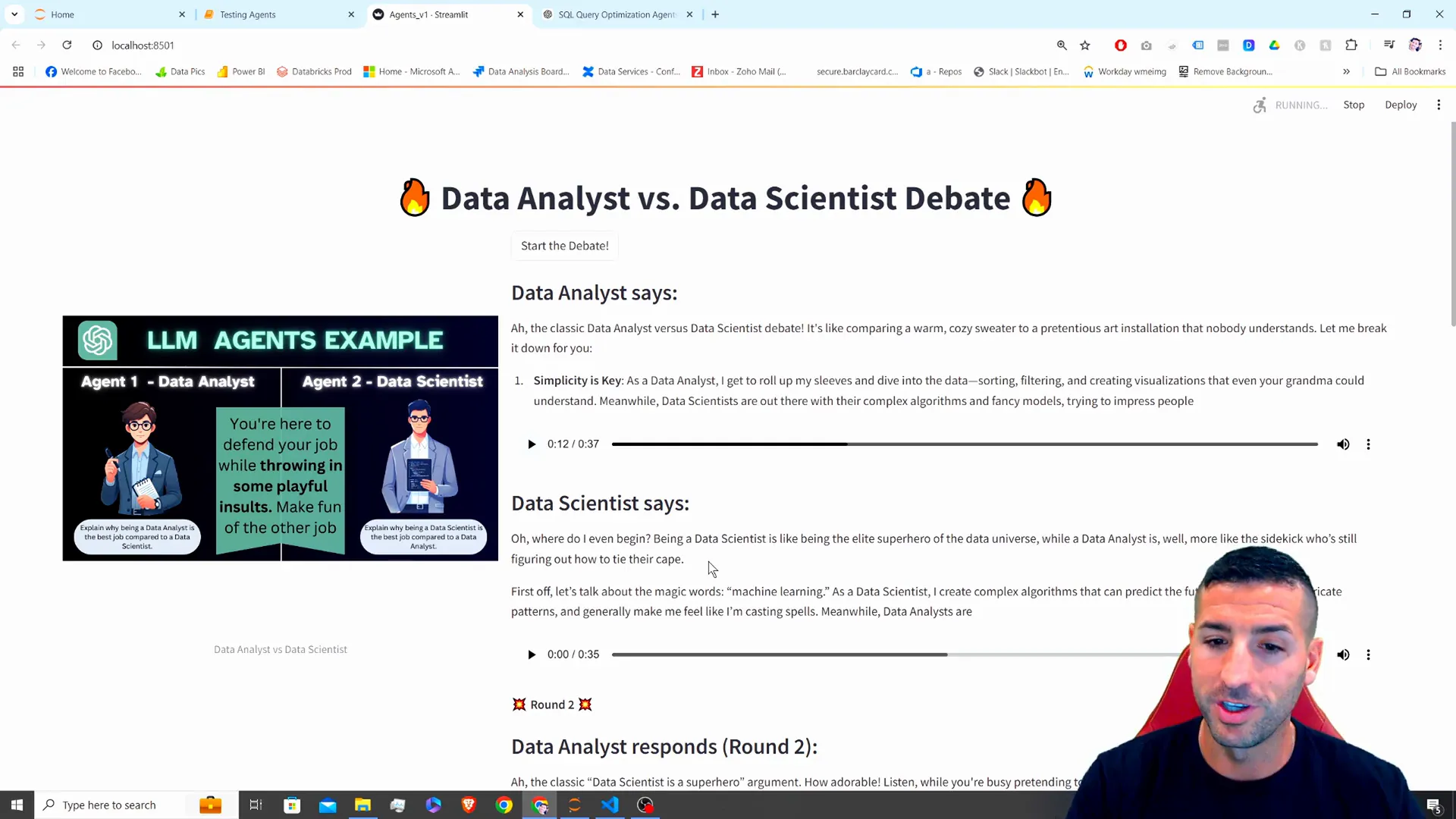